Use webpack in a project
It’s best to have webpack as a dependency in your project, through this you can choose a local webpack version and will not be forced to use the single global one.
Add a package.json
configuration file or npm
with:
$ npm init
`</pre>
The answers to the question are not so important if you don't want to publish your project to npm.
Install and add `webpack` to the `package.json` with:
<pre>`$ npm install --save-dev webpack
`</pre>
### Create a modular JavaScript Project
Let's create some modules in JavaScript, using the CommonJS syntax:
#### cat.js
<pre>`var cats = ['dave','henry','martha'];
module.exports = cats;
`</pre>
#### app.js(Entry Point)
<pre>`var cats = require('cats.js');
console.log(cats);
`</pre>
The **Entry Point** is where your application will start, and where webpack will start tracking dependencies between modules.
Give webpack the entry point(app.js) and specify an output file(app.bundle.js):
<pre>`webpack ./app.js app.bundle.js
`</pre>
webpack will read and analyze the entry point and its dependencies(including transitive dependencies). Then it will bundle them into `app.bundle.js`.
Now your bundle is ready to run. Run `node app.bundle.js` and marvel in your abundance of cats.
To gain full access to webpack's flexibility, we need to create a "configuration file"
### Project Structure
We will put the source files in **src**, and bundled files in **dist**.
The final project structure will look like this:
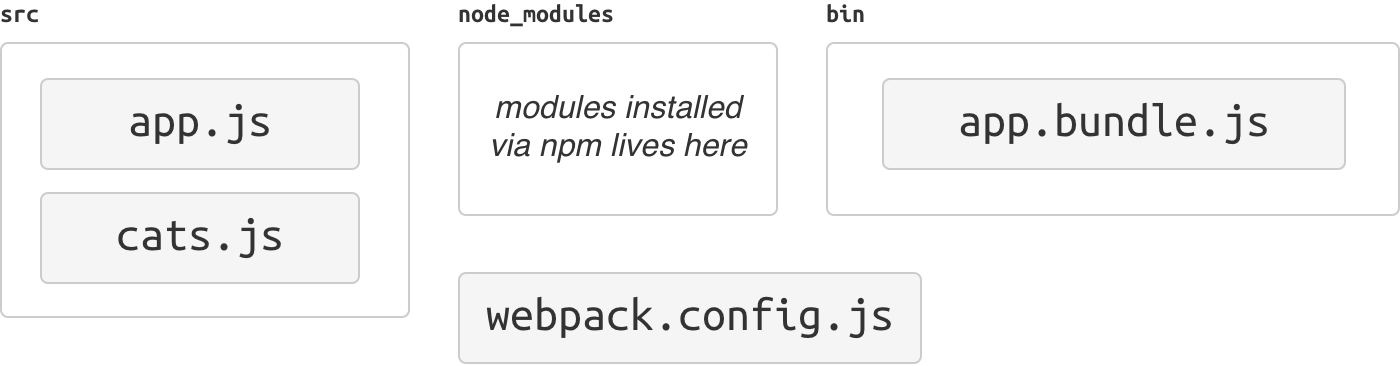
Create
webpack.config.js
`module.exports = { entry: './src/app.js', output: { path: './dist', filename: '[name].bundle.js' } }; `
A webpack configuration file is a CommonJS-Style module. So you can run any kind of code here, as long as a configuration object is exported out of its module.
Using loaders
Webpack only supports JavaScript modules natively, but most people will be using a transpiler for ES2015, CoffeeScript, TypeScript, etc. They can be used in webpack by using loaders.
Loaders are special modules webpack uses to ‘load’ other modules(written in another language) into JavaScript(that webpack understands).using
babel-loader
1. Install Babel and the presets:`npm install --save-dev babel-loader `
Configure Babel to use there presets by add
.babelrc
`{'presets':['es2015']} `
Modify
webpack.config.js
to process all.js
files usingbabel-loader
.`module.exports = { entry: './src/app.js', output: { path: './dist', filename: '[name].bundle.js' }, module: { loaders: [ {test: /\.js$/,exclude: /node_modules/, loader: 'babel-loader',} ] } } `
We are excluding
node_modules
here because otherwise all external libraries will also go through Babel, slowing down compilation.
4. Install the libraries you want to use`npm install --save jquery babel-polyfill `
We are using
--save
instead of--save-dev
this time, as there libraries will be used in runtime.-dev
means the libraries only used during development.Edit
src/app.js
`import 'babel-polyfill'; import cats from './cats'; import $ from 'jquery'; $('<h1>Cats</h1>').appendTo('body'); const ul = $('<ul></ul>').appendTo('body'); for (const cat of cats) { $('<li></li>').text(cat).appendTo(ul); }
The Uglify plugin is included with webpack so you don't need to add additional modules, but this may not always be the case. ```1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
286\. Using Plugins
An example would be minifying your file so that the client can load if faster. This can be done with **plugins**. We'll add the uglify plugin to our configuration:
const webwebpack = require('webpack');
module.exports = {
entry: './src/app.js',
output: {
path: './dist',
filename: '[name].bundle.js'
},
module: {
loaders: [
{test: /.jsx?$/, exclude: /node_modules/,loader: 'babel-loader'}
]
},
plugins: [
new webpack.optimize.UglifyJsPlugin({
compress: {
warning:false,
},
output: {
comments: false,
},
}),
]
}